Date Picker and Time
Picker dialogs are the easiest way to allow user to pick the date/time . These
are frequently used in form widget of commercial apps like makemytrip ,
bookmyshow etc.
Here in my example I have taken two editText where date and
time will be filled using Date/time Picker dialogs. You can use system image to
click and pop-up dialog box.
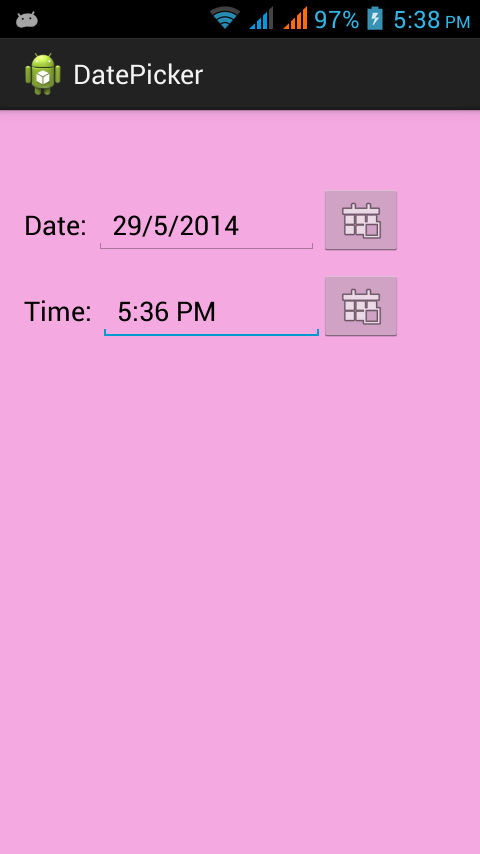
Step-1: Crate user interface , add editText and imageButtons
. Copy and paste the following codes:
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity"
android:background="#F5A9E1" >
<TextView
android:id="@+id/tvDate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginTop="48dp"
android:text="Date: "
android:textAppearance="?android:attr/textAppearanceMedium"
/>
<EditText
android:id="@+id/etDate"
android:layout_width="150dp"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/tvDate"
android:layout_alignBottom="@+id/tvDate"
android:layout_toRightOf="@+id/tvDate"
android:ems="10" />
<ImageButton
android:id="@+id/imageDate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/etDate"
android:layout_toRightOf="@+id/etDate"
android:src="@android:drawable/ic_menu_today" />
<TextView
android:id="@+id/tvTime"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/tvDate"
android:layout_below="@+id/imageDate"
android:layout_marginTop="24dp"
android:text="Time: "
android:textAppearance="?android:attr/textAppearanceMedium"
/>
<EditText
android:id="@+id/etTime"
android:layout_width="150dp"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/tvTime"
android:layout_alignBottom="@+id/tvTime"
android:layout_toRightOf="@+id/tvTime"
android:ems="10" >
<requestFocus />
</EditText>
<ImageButton
android:id="@+id/imageTime"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/etTime"
android:layout_toRightOf="@+id/etDate"
android:src="@android:drawable/ic_menu_today" />
</RelativeLayout>
Step-2: Initialize
the dialog variables as
public static final int DTPKR = 1;
public static final int TMPKR =
2;
Step-3: Inside onclickListener() call the dialog box
showDialog(DTPKR);
Step-4: Create dialog
box by defining onCreateDialog(int id)
protected Dialog
onCreateDialog(int id) {
switch (id) {
case DTPKR:
return new DatePickerDialog(this,lisDate,mYear,mMonth,mDay);
case TMPKR:
return new TimePickerDialog(this,lisTime,mHour,mMin,false);
}
return null;
}
Step-5: Set editText value form dialog box using OnDateSetListener.
//setting date and updating editText
DatePickerDialog.OnDateSetListener lisDate =
DatePickerDialog.OnDateSetListener(){
public void onDateSet(DatePicker
view, int year, int monthOfYear,int dayOfMonth) {
mYear = year;
mMonth = monthOfYear;
mDay = dayOfMonth;
etDate.setText(new StringBuilder() .append(mDay).append("/").append(mMonth+1).append("/").append(mYear));
getCurrentDate();
}
};
Do same the for timePicker dialog also.
Complete Code:
MainActivity.java
package
com.allandroidcodes.datepicker;
import java.util.Calendar;
import android.os.Bundle;
import
android.app.Activity;
import
android.app.DatePickerDialog;
import android.app.Dialog;
import
android.app.TimePickerDialog;
import android.view.Menu;
import android.view.View;
import
android.view.View.OnClickListener;
import android.view.ViewGroup;
import android.widget.Button;
import
android.widget.DatePicker;
import
android.widget.EditText;
import
android.widget.ImageButton;
import
android.widget.TimePicker;
public class MainActivity extends Activity {
//Declare variables
EditText
etDate,etTime;
ImageButton
imageDate,imageTime;
private int mMonth,mYear,mDay,mHour,mMin;
//Declare constants
public static final int DTPKR = 1;
public static final int TMPKR = 2;
protected void onCreate(Bundle
savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Setting variables
id
etDate=(EditText)
findViewById(R.id.etDate);
etTime=(EditText)
findViewById(R.id.etTime);
imageDate=(ImageButton)
findViewById(R.id.imageDate);
imageTime=(ImageButton)
findViewById(R.id.imageTime);
//Get current
system date and time
getCurrentDate();
getCurrentTime();
//show date picker
on imageButton click
imageDate.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
}
});
//show time picker
on imageButton click
imageTime.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
}
});
}
// Getting
the current date and time into DatePicker dialog
public void getCurrentDate(){
final Calendar c = Calendar.getInstance();
mYear = c.get(Calendar.YEAR);
mMonth = c.get(Calendar.MONTH);
mDay = c.get(Calendar.DAY_OF_MONTH);
}
public void getCurrentTime(){
final Calendar c = Calendar.getInstance();
mHour = c.get(Calendar.HOUR_OF_DAY);
mMin = c.get(Calendar.MINUTE);
}
//Creating dialogs
protected Dialog
onCreateDialog(int id) {
switch (id) {
case DTPKR:
return new DatePickerDialog(this,lisDate, mYear, mMonth, mDay);
case TMPKR:
return new TimePickerDialog(this,lisTime,mHour, mMin, false);
}
return null;
}
//setting date and
updating editText
DatePickerDialog.OnDateSetListener lisDate = new
DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker
view, int year, int monthOfYear,int dayOfMonth) {
mYear = year;
mMonth = monthOfYear;
mDay = dayOfMonth;
etDate.setText(new StringBuilder()
.append(mDay).append("/").append(mMonth+1).append("/").append(mYear));
getCurrentDate();
}
};
//setting time and updating editText
TimePickerDialog.OnTimeSetListener lisTime=new TimePickerDialog.OnTimeSetListener() {
@Override
public void onTimeSet(TimePicker
view, int hourOfDay, int minute) {
mHour=hourOfDay;
mMin=minute;
String AM_PM ;
if(hourOfDay < 12) {
AM_PM = "AM";
} else {
AM_PM = "PM";
mHour=mHour-12;
}
etTime.setText(mHour+":"+mMin+" "+AM_PM);
getCurrentDate();
}
};
}
Step-6: Run the project . You can see your output screens as
below:
Please comment for any doubt and suggestions. Thank You !!